00:00:00 introduction
00:00:18 example 1
00:03:58 example 2
00:07:37 conclusion
// forEach() = method used to iterate over the elements
// of an array and apply a specified function (callback)
// to each element
// array.forEach(callback)
// element, index, array are provided
// -------------- EXAMPLE 1 --------------
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(cube);
numbers.forEach(display);
function double(element, index, array){
array[index] = element * 2;
}
function triple(element, index, array){
array[index] = element * 3;
}
function square(element, index, array){
array[index] = Math.pow(element, 2);
}
function cube(element, index, array){
array[index] = Math.pow(element, 3);
}
function display(element){
console.log(element);
}
// -------------- EXAMPLE 2 --------------
let fruits = ["apple", "orange", "banana", "coconut"];
fruits.forEach(capitalize);
fruits.forEach(display);
function upperCase(element, index, array){
array[index] = element.toUpperCase();
}
function lowercase(element, index, array){
array[index] = element.toLowerCase();
}
function capitalize(element, index, array){
array[index] = element.charAt(0).toUpperCase() + element.slice(1);
}
/*
function display(element){
console.log(element);
}
*/
00:00:18 example 1
00:03:58 example 2
00:07:37 conclusion
// forEach() = method used to iterate over the elements
// of an array and apply a specified function (callback)
// to each element
// array.forEach(callback)
// element, index, array are provided
// -------------- EXAMPLE 1 --------------
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(cube);
numbers.forEach(display);
function double(element, index, array){
array[index] = element * 2;
}
function triple(element, index, array){
array[index] = element * 3;
}
function square(element, index, array){
array[index] = Math.pow(element, 2);
}
function cube(element, index, array){
array[index] = Math.pow(element, 3);
}
function display(element){
console.log(element);
}
// -------------- EXAMPLE 2 --------------
let fruits = ["apple", "orange", "banana", "coconut"];
fruits.forEach(capitalize);
fruits.forEach(display);
function upperCase(element, index, array){
array[index] = element.toUpperCase();
}
function lowercase(element, index, array){
array[index] = element.toLowerCase();
}
function capitalize(element, index, array){
array[index] = element.charAt(0).toUpperCase() + element.slice(1);
}
/*
function display(element){
console.log(element);
}
*/
- Category
- Bro Code
- Tags
- JavaScript forEach, JavaScript tutorial, JavaScript course
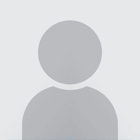
Be the first to comment