#java #javatutorial #javacourse
public class Main {
public static void main(String[] args) {
// Composition = Represents a "part-of" relationship between objects.
// For example, an Engine is "part of" a Car.
// Allows complex objects to be constructed from smaller objects.
Car car = new Car("Corvette", 2025, "V8");
System.out.println(car.model);
System.out.println(car.year);
System.out.println(car.engine.type);
car.start();
}
}
public class Car {
String model;
int year;
Engine engine;
Car(String model, int year, String engineType){
this.model = model;
this.year = year;
this.engine = new Engine(engineType);
}
void start(){
this.engine.start();
System.out.println("The " + this.model + " is running");
}
}
public class Engine {
String type;
Engine(String type){
this.type = type;
}
void start(){
System.out.println("You start the " + this.type + " engine");
}
}
public class Main {
public static void main(String[] args) {
// Composition = Represents a "part-of" relationship between objects.
// For example, an Engine is "part of" a Car.
// Allows complex objects to be constructed from smaller objects.
Car car = new Car("Corvette", 2025, "V8");
System.out.println(car.model);
System.out.println(car.year);
System.out.println(car.engine.type);
car.start();
}
}
public class Car {
String model;
int year;
Engine engine;
Car(String model, int year, String engineType){
this.model = model;
this.year = year;
this.engine = new Engine(engineType);
}
void start(){
this.engine.start();
System.out.println("The " + this.model + " is running");
}
}
public class Engine {
String type;
Engine(String type){
this.type = type;
}
void start(){
System.out.println("You start the " + this.type + " engine");
}
}
- Category
- Bro Code
- Tags
- java tutorial, java course, java programming
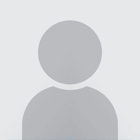
Be the first to comment